Hello Everyone!
In this tutorial we will see how to create a simple Todo list API with CRUD operations along with MySQL and Spring Boot Data JPA.
What is Spring Boot?
Spring Boot is a framework which is build on top of Spring Framework. It provides an easier and faster way to set up, configure, and run both simple and web applications.
What is MySQL?
MySQL is an open-source relational database management system.
Prerequisites
1. Java 11 Installed
2. XAMPP/ WAMP Installed
3. Rest Client (Ex. Postman) Installed (To test the API)
4. Code Editor/ IDE (Ex. IntelliJ IDEA)
Setting Up the Project
Step 1 :
Unlike other frameworks, Spring Boot provides an unique online project generation tool where you can initially setup your project and download the ready made source code.
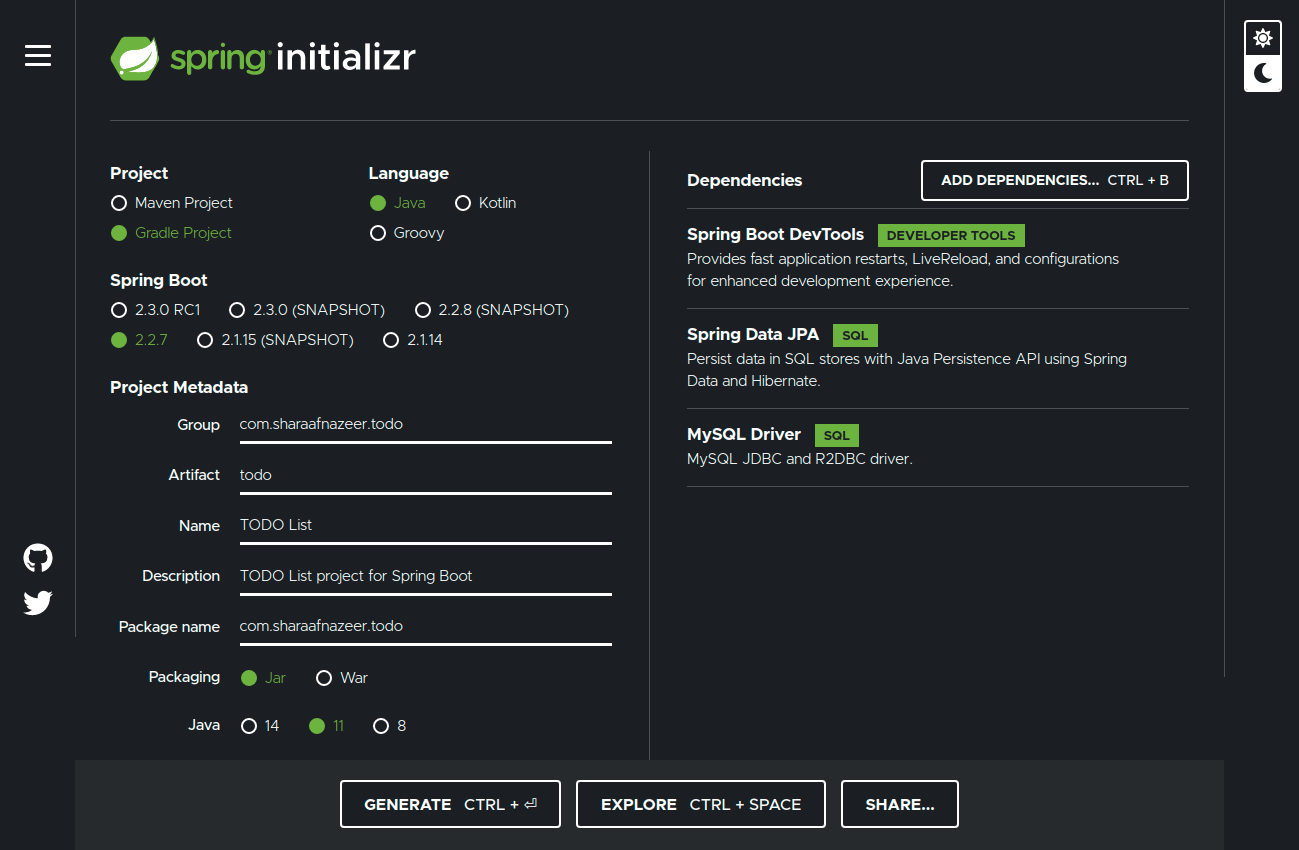
You can find the project setting via the following link
Step 2 :
Open the downloaded project with your IDE (IntelliJ IDEA is better) and sync it properly.
Project Structure
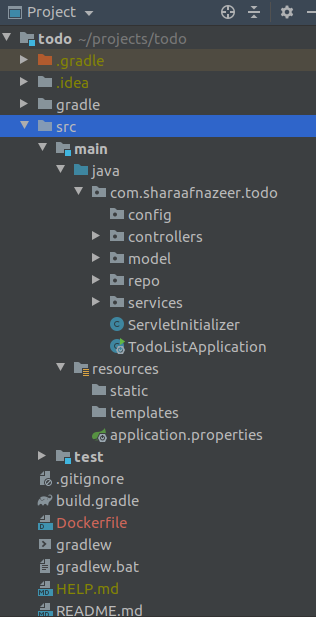
Start Coding!!
1. Creating Properties File
Here we usually give our database related configurations such as server, db name, database engine type etc.
spring.jpa.hibernate.ddl-auto=update tells our ORM to automatically update the database when a new entity is created or existing entity is modified
2. Creating Entity Class
In order to perform Database operations, we need to create entity class to map out database column with Java class attributes
Since we are using Spring Data JPA along with Hibernate, above class will create the required table with respective fields in the database.
3. Creating Repository Interface
Spring Data JPA provides built-in methods for performing basic CRUD operations and we don’t need to write any query method. Therefore, Spring generates the respective queries from your method to perform database operations.
So basically we only need to extend our TodoRepository interface with JpaRepository interface and we don’t need to write any query method inside this repository.
4. Creating Service Interface & Implementation Class
Service Components are the class file which contains @Service annotation. The service class is used generally if we want to perform some business logic or conversion to different data.
Initially we can create an Interface which contains store, get and delete methods using the code as shown below
The following code will let you to create a class which implements the TodoService interface with @Service annotation and write the business logic to store, retrieve, delete and updates the product.
In order to do that, initially we need to inject out TodoRepository interface within out service class
Since JpaRepository provides built in methods, we can use those methods to interact with our database.
5. Creating REST Controller
Now we will call our CRUD operations with a REST API so that any client can consume our REST service and do CRUD operations. Here we @Autowired the TodoService interface and called the methods.
6. Modifying Main Class
We also need to tell Spring where our repository interface is located. So we need to annotate the location with @EnableJpaRepositories
Testing the Application
Run the main class to start the application.
1. Creating Todo
Create Todo using REST client as shown below
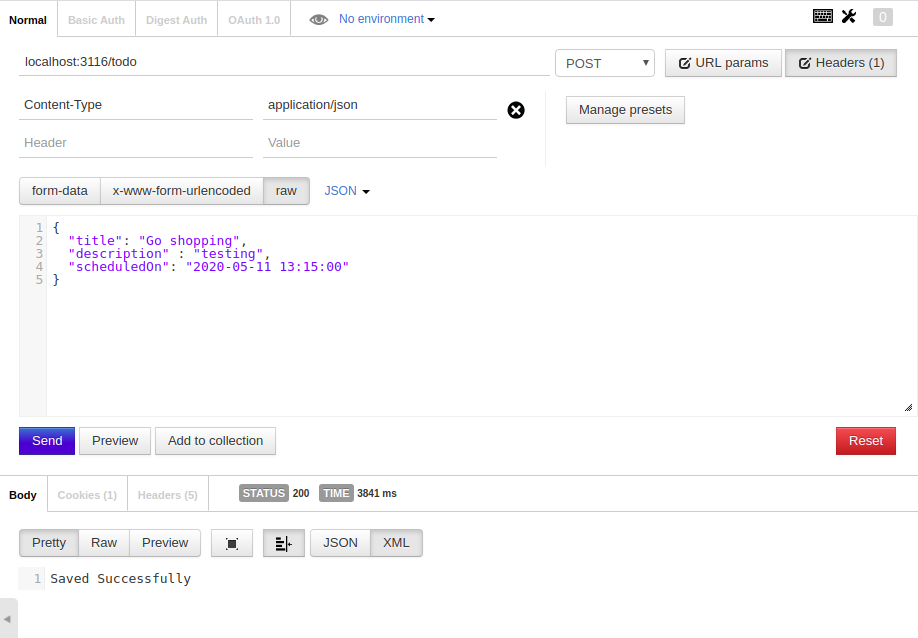
2. Getting all Todo List
Now we will try to retrieve all available Todo
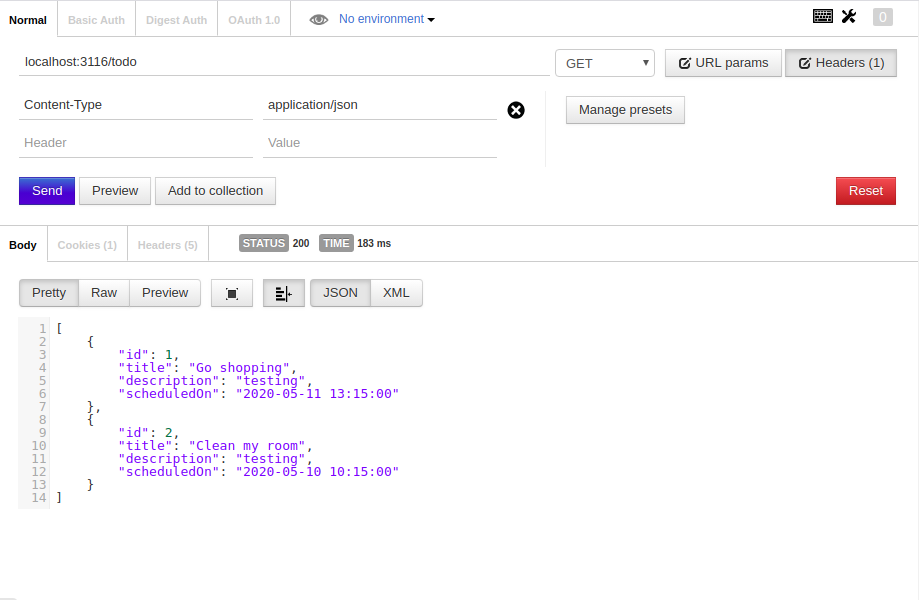
2. Updating a Todo
Now we will try to update Todo with id = 1
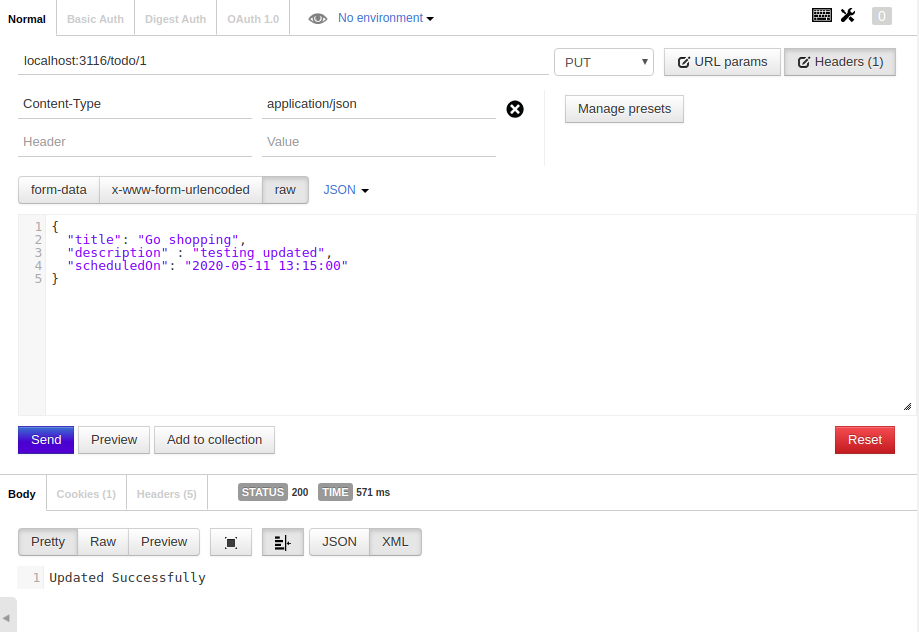
3. Getting a Todo
Now we will try to retrieve Todo with id = 2
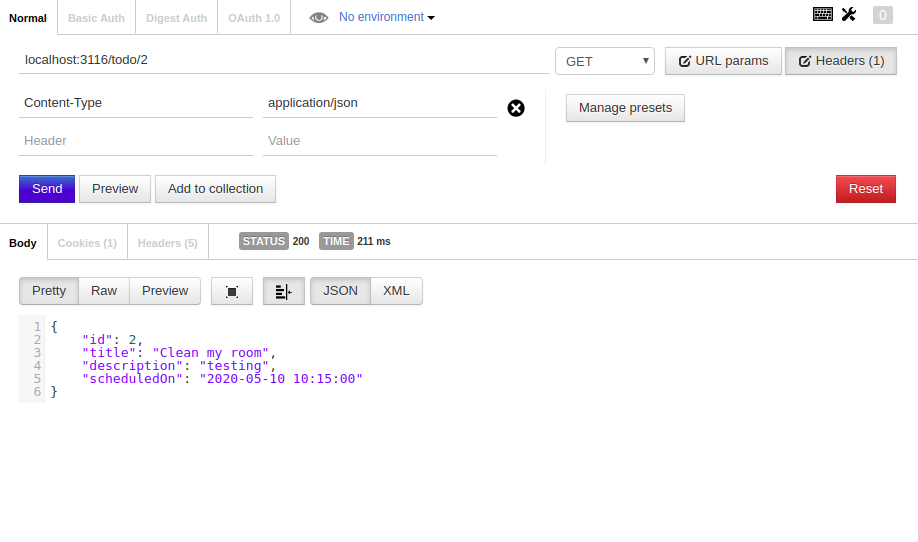
4. Deleting a Todo
Now we will try to delete Todo with id = 1
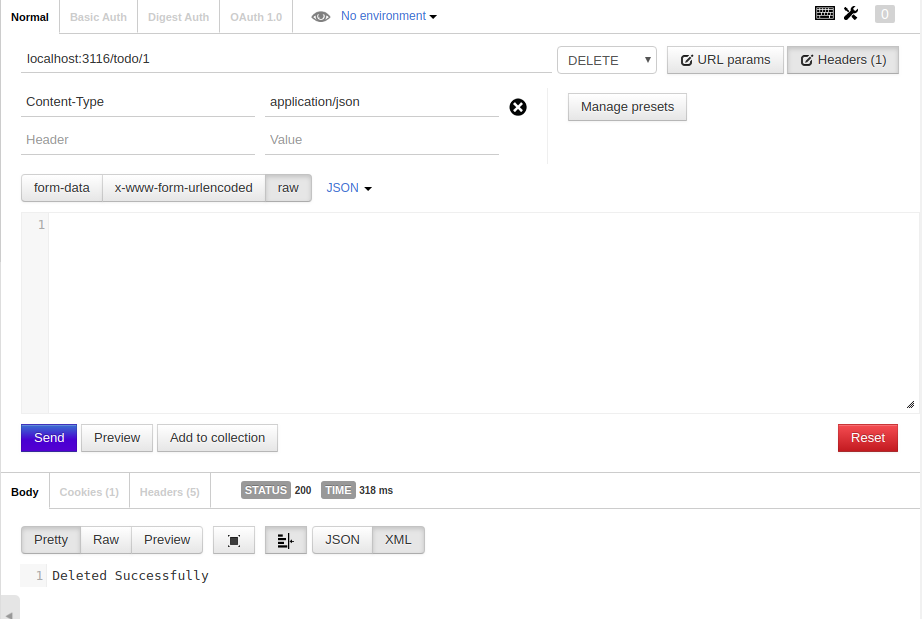
That’s it. Now we have successfully implemented a REST API using Spring Boot. In the mean time you can cross check with the Database whether it is getting updated correctly
Complete project can be found here
Thanks for Reading!!